Routing in Next.js
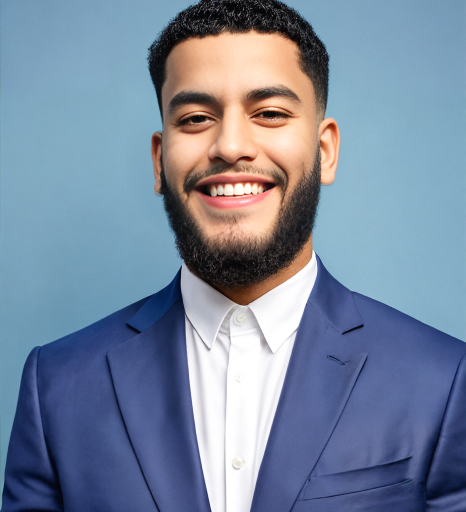
Taha Lakrati
Sep 3, 2023
If you're dipping your toes into the realm of Next.js, understanding its routing system can make your development journey much smoother. Today, we'll explore the various routing options in this framework.
The basics of Routing in Next.JS
Routing refers to how we decide what components or pages to display based on the URL. With Next.js, you don't need to wrestle with additional libraries or configurations. Here's how:
Add a file inside the pages directory, say `about.js`
Navigate to `yourwebsite.com/about`
Next.js will automatically render the component or page you defined in `about.js`
// pages/about.js
const About = () => {
return <div>About us</div>;
}
export default About;
Just visit `yourwebsite.com/about`, and you'll see "About us".
Dynamic Routing
For data-driven routes, like for blog posts or user profiles, Next.js introduces dynamic routing.
Create a dynamic route using square brackets `[ ]` in your filename or folder name inside the pages directory.
// pages/posts/[postId].js
const Post = ({ post }) => {
return <div>{post.title}</div>;
}
// Fetch post data here...
export default Post;
Here, `[postId]` is a placeholder. It will match any value, rendering the appropriate post based on the URL.
Server-side Data Fetching
Beyond basic client-side navigation, Next.js excels with its server-side data fetching. This fetches data before rendering the page, perfect for up-to-date content and SEO. For instance:
// pages/user.js
const User = ({ userData }) => {
return <div>{userData.name}'s profile</div>;
}
export const getServerSideProps = async (context) => {
// Fetch data from an API or a database
const response = await fetch('https://api.example.com/user');
const userData = await response.json();
// Return the data as props
return {
props: {
userData
}
};
}
export default User;
In this example, every time the user page is accessed, Next.js fetches the latest user data server-side, ensuring users see the most recent data without additional client-side fetches.
Summary
That's a wrap, folks. You can now efficiently use NextJS's routing system!🚀
Resources
Here are some of the resources that inspired this note:
Documentation